Development is at the very top of the frustration pyramid. Learning new things may be both enjoyable and frustrating. Unfortunately, the majority of job offers today demand that you design and construct websites, therefore being able to code at least some front-end is essential for UX/UI designers.
Let me share with you 5 CSS Tricks for Front-end Developers that have been quite helpful to me over the years as I’ve built a few websites.
1. Typing effect for text
Your website’s viewers can be engaged and kept interested in reading more by adding typewriter effects to sections of your content. Making compelling landing pages, call-to-action buttons, personal websites, and code presentations are just a few uses for the typewriter effect.
Let’s start by building the website for our typewriter demonstration. It will have a div
container for the typewritten content with the following class:
<!DOCTYPE HTML>
<html>
<head>
<title>Typing effect for text</title>
<meta charset="UTF-8" />
<style>
.typing {
height: 100px;
display: flex;
align-items: center;
justify-content: flex-start;
background: black;
color: #ffffff;
padding: 0 10px;
}
.typing-effect {
width: 22ch;
animation: typing 3s steps(22), effect 0.4s step-end infinite alternate;
white-space: nowrap;
overflow: hidden;
border-right: 3px solid;
font-family: monospace;
font-size: 1.3em;
}
@keyframes typing {
from {
width: 0;
}
}
@keyframes effect {
50% {
border-color: transparent;
}
}
</style>
</head>
<body>
<div class="typing">
<div class="typing-effect">
Hi My Name's Mo'tasem
</div>
</div>
</body>
</html>
Results:
2. Outer Glow
Box-shadow is a highly potent CSS feature. As if our HTML components were in a genuine 3D environment and could be manipulated along the z-axis to rise above the backdrop content or sink in it, it allows developers to imitate drop-shadows.
<!DOCTYPE HTML>
<html>
<head>
<title>Outer Glow</title>
<meta charset="UTF-8" />
<style>
.glow-button
{
display: flex;
justify-content: center;
align-items: center;
width: 180px;
padding: 1.5rem;
margin-top: 25px;
background: white;
color: #48abe0;
text-align: center;
cursor: pointer;
box-shadow: 0 0 50px 15px #48abe0;
font-weight: 600;
font-size: 18px;
}
.container-glow {
padding: 10rem;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
background-color: black;
color: white;
text-align: center;
}
</style>
</head>
<body>
<div class="container-glow">
<div class="glow-button">Button</div>
</div>
</body>
</html>
Results:
3. Creating Shapes
Both novice and experienced developers frequently fail to realize that shapes may be created directly in CSS. Of fact, making squares and rectangles has practically always been feasible; all you need to do is give a div a backdrop color. You may also make a wide range of triangles, trapezoids, arrows, stars, and many more shapes with CSS. In fact, there are so many more that included the code for each one would more than double the size of this post. We’ll be content with a few instances instead;
Code and outputs:
Square
<!DOCTYPE HTML>
<html>
<head>
<title>Square</title>
<meta charset="UTF-8" />
<style>
#square {
width: 100px;
height: 100px;
background: #fba200;
}
</style>
</head>
<body>
<div id="square"></div>
</body>
</html>
Circle
<!DOCTYPE HTML>
<html>
<head>
<title>Circle</title>
<meta charset="UTF-8" />
<style>
#circle {
width: 100px;
height: 100px;
background: #fba200;
border-radius: 50%
}
</style>
</head>
<body>
<div id="circle"></div>
</body>
</html>
4. Color Fade on Hover
Of course, hover effects are aesthetically pleasing. When utilized appropriately, they may offer a web page a more dynamic sense, making it feel less like a static wall of text and graphics. They can also look quite beautiful. Hover effects have visual advantages, but they also serve an accessibility function by clearly indicating to the user that they are lingering over a dynamic element, such a link.
Color fade takes two CSS attributes, which makes it a little more difficult to implement than fixed table layout. The first of them has to be set to normal because it is your typical hover type. The root of your class will go in the transition property, which is the second.
<!DOCTYPE HTML>
<html>
<head>
<title>Auto-Sizing Columns</title>
<meta charset="UTF-8" />
<style>
#element {
transition: all 0.5s ease;
background: #ff9900;
color: #000000;
font-size: 20px;
font-weight: 600;
}
#element:hover {
background: yellow;
}
</style>
</head>
<body>
<div id="element">1991</div>
</body>
</html>
Results
5. Easily resize images to fit
Sometimes you’re in a bind and need to scale photographs proportionately to match a specific width. Using max width to manage this is a simple solution. Here is an illustration:
<!DOCTYPE HTML>
<html>
<head>
<title>Auto-Sizing Columns</title>
<meta charset="UTF-8" />
<style>
.img {
max-width:100%;
height:auto;
}
</style>
</head>
<body>
<img class="img" src="https://motasemodeh.com/wp-content/uploads/2022/08/business-impact.jpg">
</body>
</html>
Results
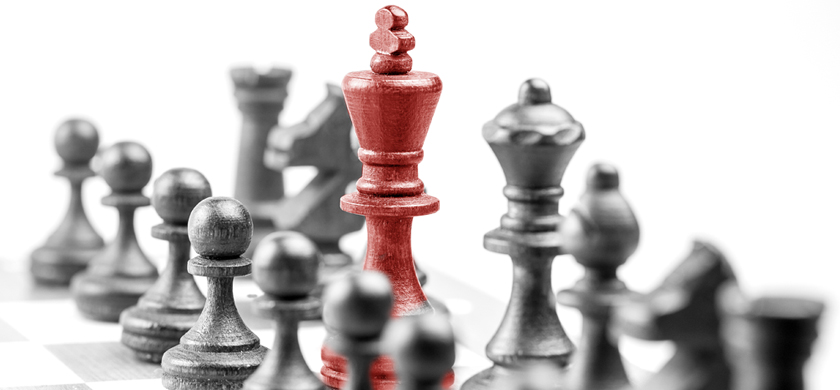